세 개 이상의 화면 만들어 전환하기
(Do it! 안드로이드앱 프로그래밍 도전과제 _08)
앱에서 사용될 수 있는 여러 화면을 구성하고
각 화면을 전환하면서 토스트로
메시지를 띄워주도록 만들어 보세요

① 로그인 화면과 메뉴 화면 그리고 세개의 서브 화면 (고객 관리 화면, 매출 관리 화면, 상품 관리 화면)을
각각 액티비티로 만듭니다.
② 로그인 화면에는 두 개의 입력상자와 하나의 버튼이 들어가도록 합니다.
③ 메뉴 화면에는 세 개의 버튼이 들어가도록 하고 각각 '고객 관리', '매출 관리', '상품 관리'라는 이름으로
표시합니다.
④ 로그인 화면의 [로그인] 버튼을 누르면 메뉴 화면으로 이동합니다. 만약 사용자 이름이나 비밀번호가
입력되어 있지 않은 상태에서 [로그인] 버튼을 누르면 토스트로 입력하라는 메시지를 보여주고 대기합니다.
⑤ 메뉴 화면의 버튼 중에서 하나를 누르면 해당 서브 화면으로 이동합니다. 메뉴 화면에 있는 [로그인] 버튼을
누르면 로그인 화면으로 이동하고 각 서브 화면에 있는 [메뉴] 버튼을 누르면 메뉴 화면으로 이동합니다.
※ 참고할 점
각 화면은 액티비티로 만들고 startActivityForResult 메서드로 새로 띄우건 finish 메서드를 사용해서
원래의 화면으로 돌아올 수 있게 합니다.
그리고 어떤 화면으로부터 보내온 응답인지 모두 확인하여 모두 확인하여 토스트 메시지로 보여줍니다.
[CODE]
<activity_main.xml>
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
<activity_login.xml>
<?xml version="1.0"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="110dp"
android:layout_gravity="center"
android:layout_margin="10dp"
android:paddingTop="50dp"
android:text="로그인하기"
android:textSize="20sp"
android:textStyle="bold" />
</LinearLayout>
<RelativeLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#aaffffff"
android:layout_centerInParent="true"
android:layout_margin="10dp">
<LinearLayout
android:layout_toLeftOf="@+id/loginButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="4dp"
android:layout_alignParentLeft="true"
android:orientation="vertical">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="4dp"
android:orientation="horizontal">
<TextView
android:id="@+id/usernameLabel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="16dp"
android:textColor="#ff222222"
android:text="사용자명 : " />
<EditText
android:id="@+id/usernameInput"
android:layout_width="170dp"
android:layout_height="wrap_content"
android:layout_alignBaseline="@id/usernameLabel"/>
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="4dp"
android:orientation="horizontal">
<TextView
android:id="@+id/passwordLabel"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="16dp"
android:textColor="#ff222222"
android:text="비밀번호 : "/>
<EditText
android:id="@+id/passwordInput"
android:layout_width="170dp"
android:layout_height="wrap_content"
android:layout_alignBaseline="@id/passwordLabel"
android:inputType="textPassword"
android:layout_below="@+id/usernameInput"/>
</LinearLayout>
</LinearLayout>
<Button
android:id="@+id/loginButton"
android:layout_width="100dp"
android:layout_height="100dp"
android:text="로그인"
android:layout_marginRight="4dp"
android:layout_centerVertical="true"
android:layout_alignParentRight="true"/>
</RelativeLayout>
</RelativeLayout>
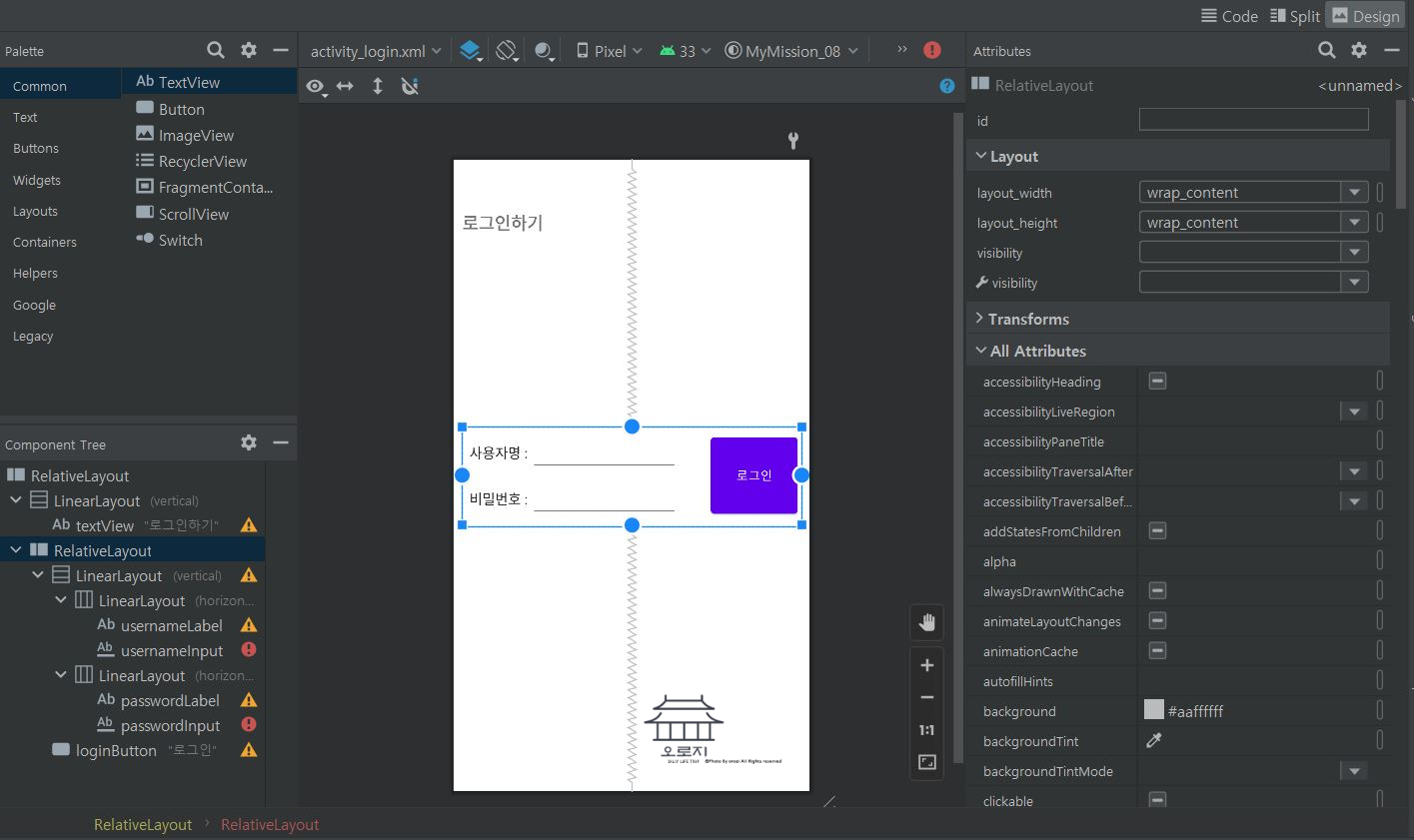
<activity_menu.xml>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="20dp">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="메인메뉴"
android:textSize="25sp"
android:textStyle="bold" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:background="#aaffffff"
android:orientation="vertical"
android:padding="20dp">
<Button
android:id="@+id/menu01Button"
android:layout_width="180dp"
android:layout_height="wrap_content"
android:text="고객관리"
android:textSize="18sp"
android:textStyle="bold" />
<Button
android:id="@+id/menu02Button"
android:layout_width="180dp"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:text="매츌관리"
android:textSize="18sp"
android:textStyle="bold" />
<Button
android:id="@+id/menu03Button"
android:layout_width="180dp"
android:layout_height="wrap_content"
android:text="상품관리"
android:layout_marginTop="30dp"
android:textSize="18sp"
android:textStyle="bold" />
<Button
android:id="@+id/backButton"
android:layout_height="wrap_content"
android:layout_width="180dp"
android:textStyle="bold"
android:textSize="18dp"
android:text="로그인 화면"
android:layout_marginTop="30dp"/>
</LinearLayout>
</RelativeLayout>
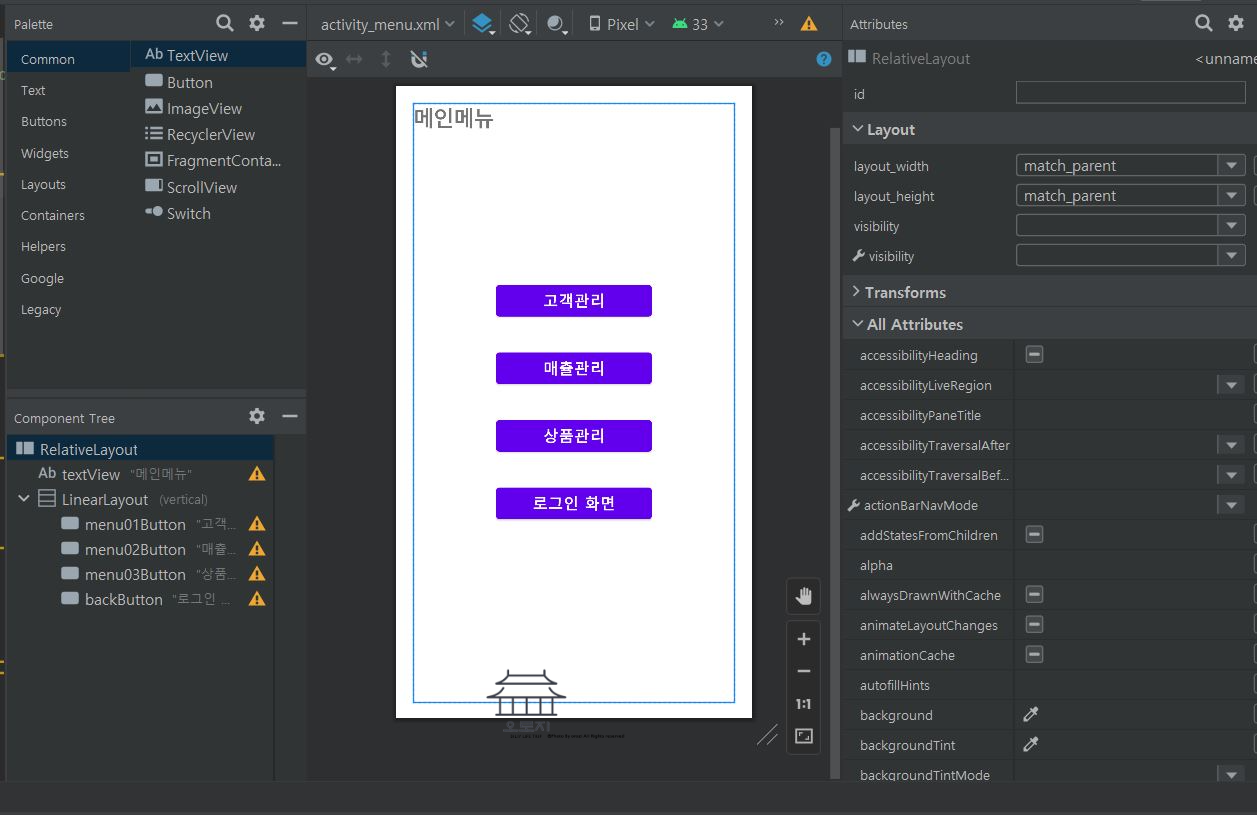
<activity_customer.xml>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#aaffffff"
android:layout_centerInParent="true"
android:padding="20dp"
android:layout_margin="20dp"
android:orientation="vertical">
<TextView
android:id="@+id/titleText"
android:layout_width="180dp"
android:layout_height="wrap_content"
android:textColor="#ff333333"
android:textStyle="bold"
android:textSize="18dp"
android:text="고객 관리"
android:gravity="center_horizontal" />
<Button
android:id="@+id/backButton"
android:layout_width="180dp"
android:layout_height="wrap_content"
android:textStyle="bold"
android:textSize="18dp"
android:text="메뉴 화면"
android:layout_marginTop="30dp"/>
</LinearLayout>
</RelativeLayout>
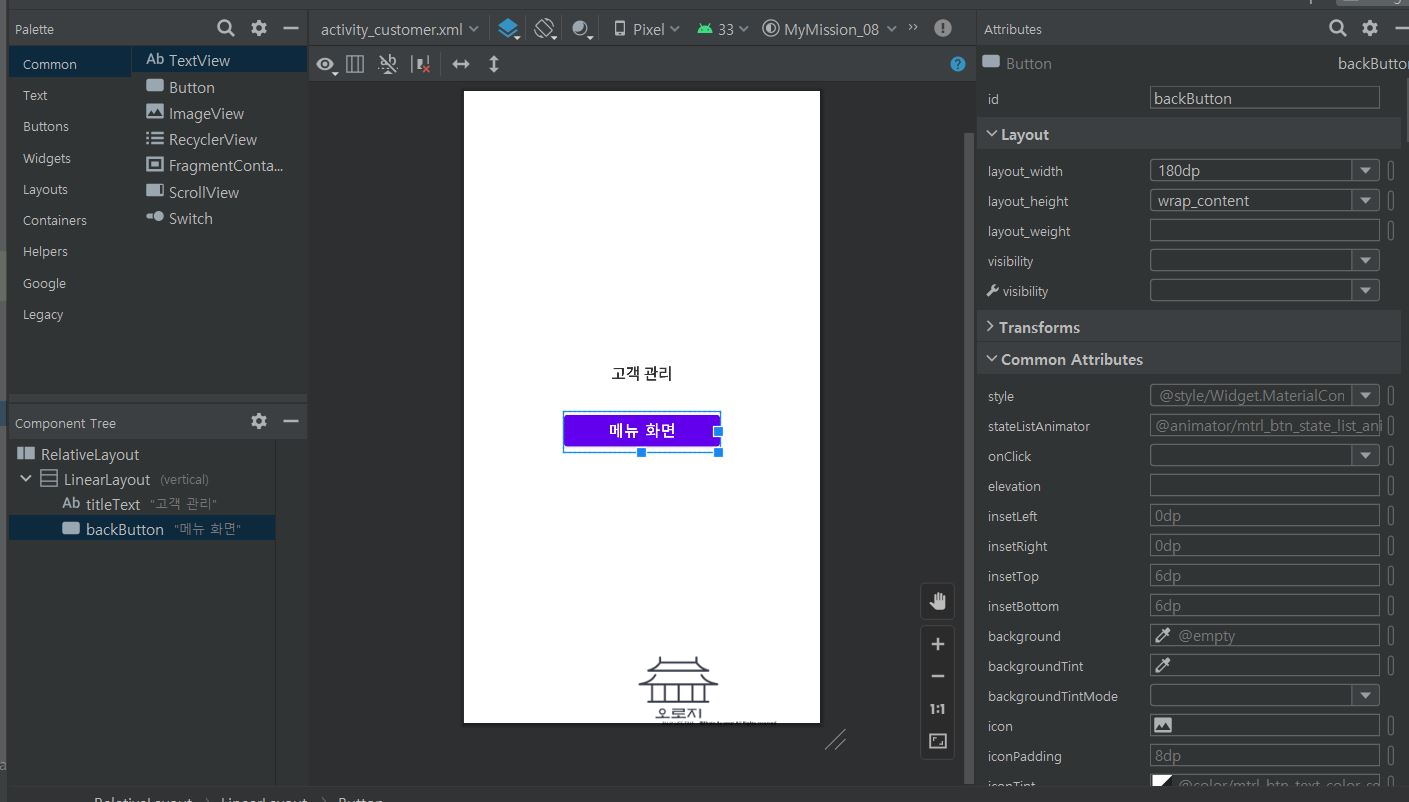
<activity_product.xml>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#aaffffff"
android:layout_centerInParent="true"
android:padding="20dp"
android:layout_margin="20dp"
android:orientation="vertical">
<TextView
android:id="@+id/titleText"
android:layout_width="180dp"
android:layout_height="wrap_content"
android:textColor="#ff333333"
android:textStyle="bold"
android:textSize="18dp"
android:text="상품 관리"
android:gravity="center_horizontal" />
<Button
android:id="@+id/backButton"
android:layout_width="180dp"
android:layout_height="wrap_content"
android:textStyle="bold"
android:textSize="18dp"
android:text="메뉴 화면"
android:layout_marginTop="30dp"/>
</LinearLayout>
</RelativeLayout>
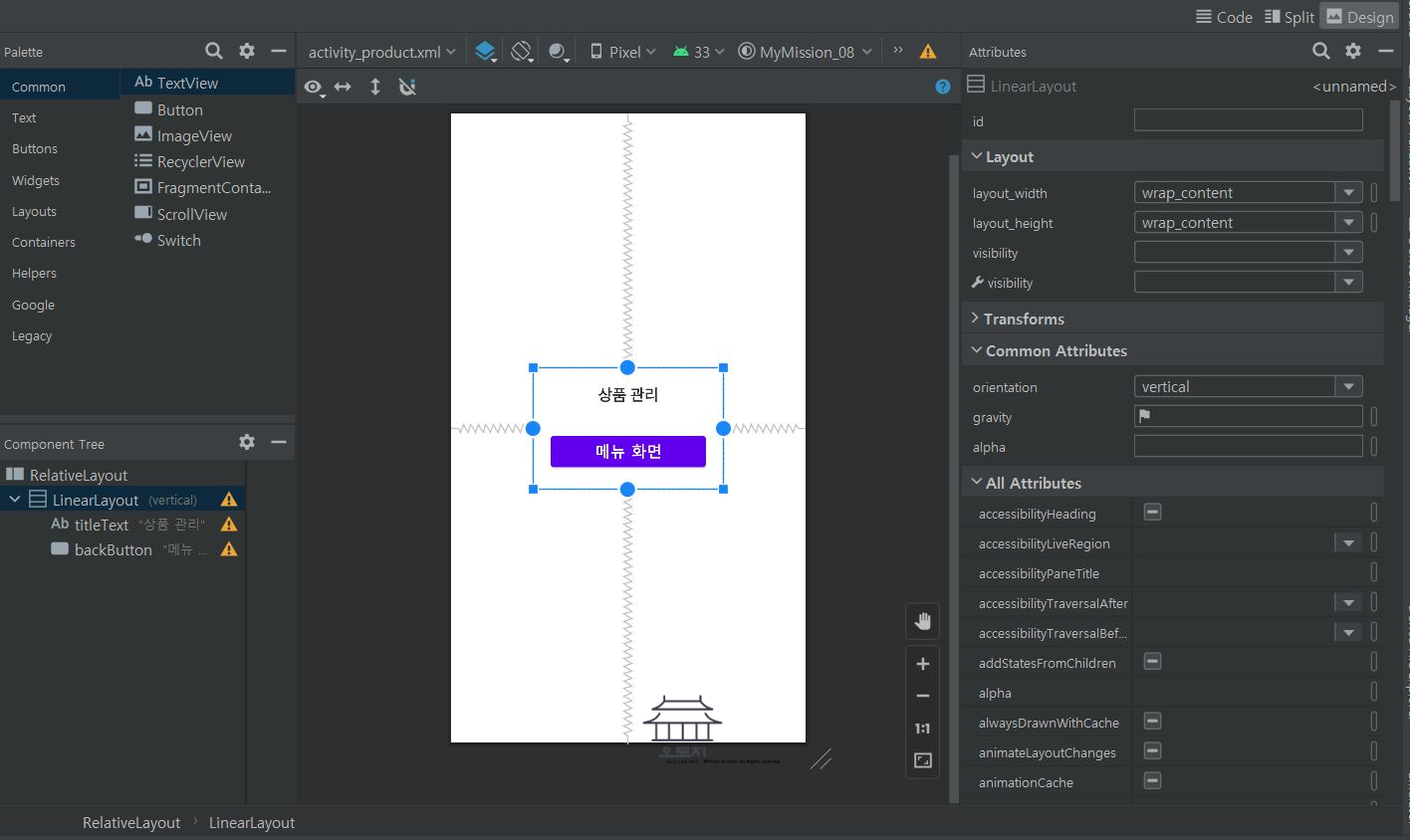
<activity_revenue.xml>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#aaffffff"
android:layout_centerInParent="true"
android:padding="20dp"
android:layout_margin="20dp"
android:orientation="vertical">
<TextView
android:id="@+id/titleText"
android:layout_width="180dp"
android:layout_height="wrap_content"
android:textColor="#ff333333"
android:textStyle="bold"
android:textSize="18dp"
android:text="매출 관리"
android:gravity="center_horizontal" />
<Button
android:id="@+id/backButton"
android:layout_width="180dp"
android:layout_height="wrap_content"
android:textStyle="bold"
android:textSize="18dp"
android:text="메뉴 화면"
android:layout_marginTop="30dp"/>
</LinearLayout>
</RelativeLayout>
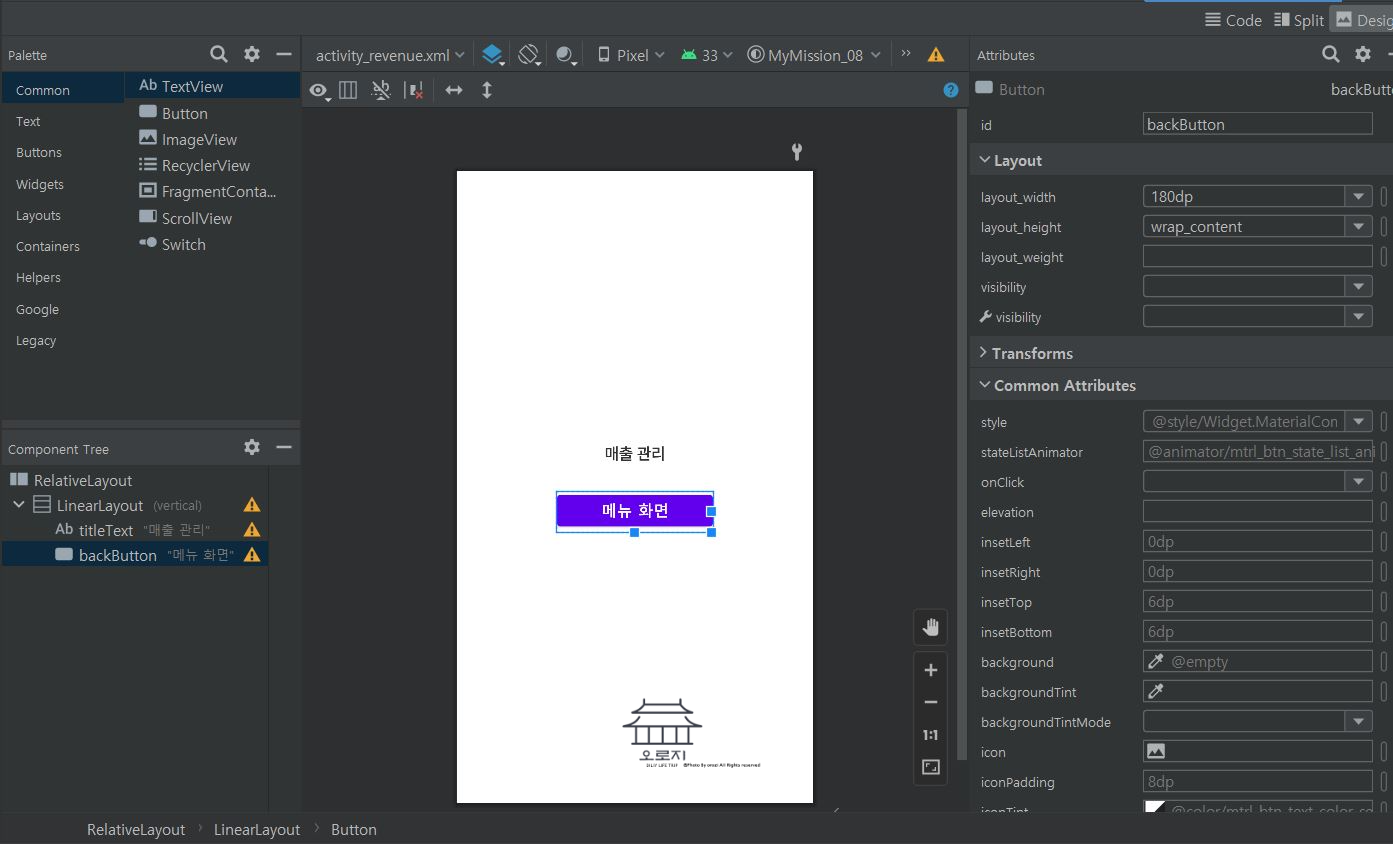
<MainActivity.java>
package com.example.mission_08;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
}
<LoginActivity.java>
package com.example.mission_08;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class LoginActivity extends AppCompatActivity {
public static final int REQUEST_CODE_MENU = 101;
EditText usernameInput;
EditText passwordInput;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
Button loginButton = findViewById(R.id.loginButton);
loginButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String username = usernameInput.getText().toString();
String password = passwordInput.getText().toString();
Intent intent = new Intent(getApplicationContext(), MenuActivity.class);
intent.putExtra("username", username);
intent.putExtra("password", password);
startActivityForResult(intent, REQUEST_CODE_MENU);
}
});
usernameInput = findViewById(R.id.usernameInput);
passwordInput = findViewById(R.id.passwordInput);
}
protected void onActivityResult(int requestCode, int resultCode, Intent intent) {
super.onActivityResult(requestCode, resultCode, intent);
if (requestCode == REQUEST_CODE_MENU) {
if (intent != null) {
String menu = intent.getStringExtra("menu");
String message = intent.getStringExtra("message");
Toast toast = Toast.makeText(getBaseContext(), "result code : " + resultCode + ", " +
"menu : " + menu + ", message : " + message, Toast.LENGTH_LONG);
toast.show();
}
}
}
}
<MenuActivity.java>
package com.example.mission_08;
import static com.example.mission_08.R.id.menu01Button;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MenuActivity extends AppCompatActivity {
public static final int REQUEST_CODE_CUSTOMER = 201;
public static final int REQUEST_CODE_REVENUE =202 ;
public static final int REQUEST_CODE_PRODUCT = 203;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_menu);
// process received intent
Intent receivedIntent = getIntent();
String username = receivedIntent.getStringExtra("username");
String password = receivedIntent.getStringExtra("password");
Toast.makeText(this, "username : " + username + ", password : " + password, Toast.LENGTH_LONG).show();
Button backButton = findViewById(R.id.backButton);
backButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent resultIntent = new Intent();
resultIntent.putExtra("message", "result message is OK!");
setResult(Activity.RESULT_OK, resultIntent);
finish();
}
});
Button menu01Button = findViewById(R.id.menu01Button);
menu01Button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent intent = new Intent(getApplicationContext(), CustomerActivity.class);
intent.putExtra("titleMsg", "고객관리 화면");
startActivityForResult(intent, REQUEST_CODE_CUSTOMER);
}
});
Button menu02Button = findViewById(R.id.menu02Button);
menu02Button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent intent = new Intent(getApplicationContext(), RevenueActivity.class);
intent.putExtra("titleMsg", "매출관리 화면");
startActivityForResult(intent, REQUEST_CODE_REVENUE);
}
});
Button menu03Button = findViewById(R.id.menu03Button);
menu03Button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent intent = new Intent(getApplicationContext(), ProductActivity.class);
intent.putExtra("titleMsg", "상품관리 화면");
startActivityForResult(intent, REQUEST_CODE_PRODUCT);
}
});
}
protected void onActivityResult(int requestCode, int resultCode, Intent intent) {
super.onActivityResult(requestCode, resultCode, intent);
if (intent != null) {
if (requestCode == REQUEST_CODE_CUSTOMER) {
String message = intent.getStringExtra("message");
if (message != null) {
showToast("고객관리 응답, result code : " + resultCode + ", message : " + message);
}
} else if (requestCode == REQUEST_CODE_REVENUE) {
String message = intent.getStringExtra("message");
if (message != null) {
showToast("매출관리 응답, result code : " + resultCode + ", message : " + message);
}
} else if (requestCode == REQUEST_CODE_PRODUCT) {
String message = intent.getStringExtra("message");
if (message != null) {
showToast("상품관리 응답, result code : " + resultCode + ", message : " + message);
}
}
}
}
private void showToast(String s) {
Toast.makeText(getApplicationContext(), "message", Toast.LENGTH_SHORT).show();
}
}
<CustometActivity.java>
package com.example.mission_08;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class CustomerActivity extends AppCompatActivity {
TextView titleText;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_customer);
titleText = findViewById(R.id.titleText);
// process received intent
Intent receivedIntent = getIntent();
String titleMsg = receivedIntent.getStringExtra("titleMsg");
Toast.makeText(this, "titleMsg : " + titleMsg, Toast.LENGTH_LONG).show();
if (titleText != null) {
titleText.setText(titleMsg);
}
Button backButton = findViewById(R.id.backButton);
backButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent resultIntent = new Intent();
resultIntent.putExtra("message", "result message is OK!");
setResult(Activity.RESULT_OK, resultIntent);
finish();
}
});
}
}
<ProductActivity.java>
import android.annotation.SuppressLint;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class ProductActivity extends AppCompatActivity {
TextView titleText;
@SuppressLint("MissingInflatedId")
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_product);
titleText = findViewById(R.id.titleText);
// process received intent
Intent receivedIntent = getIntent();
String titleMsg = receivedIntent.getStringExtra("titleMsg");
Toast.makeText(this, "titleMsg : " + titleMsg, Toast.LENGTH_LONG).show();
if (titleText != null) {
titleText.setText(titleMsg);
}
Button backButton = findViewById(R.id.backButton);
backButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent resultIntent = new Intent();
resultIntent.putExtra("message", "result message is OK!");
setResult(Activity.RESULT_OK, resultIntent);
finish();
}
});
}
}
<RevenueActivity.java>
public class RevenueActivity extends AppCompatActivity {
TextView titleText;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_revenue);
titleText = (TextView) findViewById(R.id.titleText);
// process received intent
Intent receivedIntent = getIntent();
String titleMsg = receivedIntent.getStringExtra("titleMsg");
Toast.makeText(this, "titleMsg : " + titleMsg, Toast.LENGTH_LONG).show();
if (titleText != null) {
titleText.setText(titleMsg);
}
Button backButton = (Button) findViewById(R.id.backButton);
backButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
Intent resultIntent = new Intent();
resultIntent.putExtra("message", "result message is OK!");
setResult(Activity.RESULT_OK, resultIntent);
finish();
}
});
}
}
<Manifest.xml>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.MyMission_08"
tools:targetApi="31">
<activity android:label="@string/app_name"
android:name=".LoginActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
<activity android:name=".MenuActivity"/>
<activity android:name=".CustomerActivity"/>
<activity android:name=".ProductActivity"/>
<activity android:name=".RevenueActivity"/>
</application>
</manifest>
<실행결과 화면>
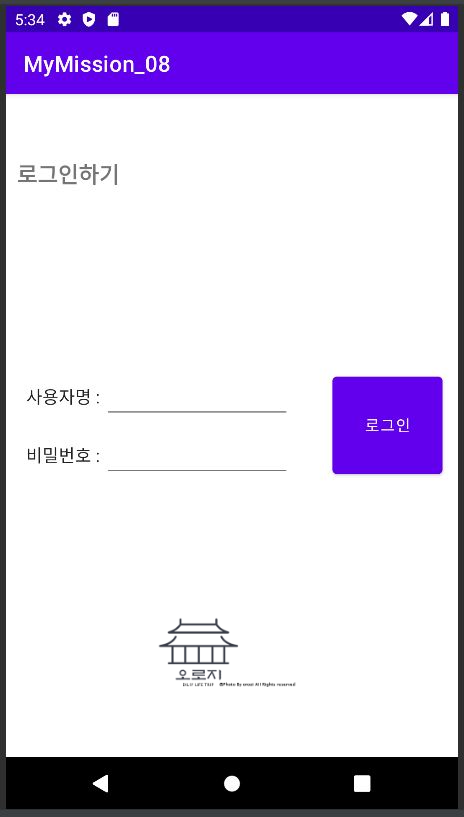
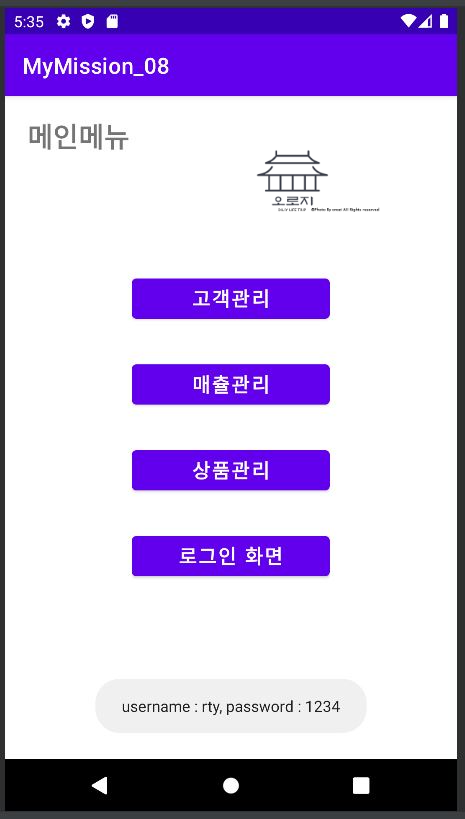
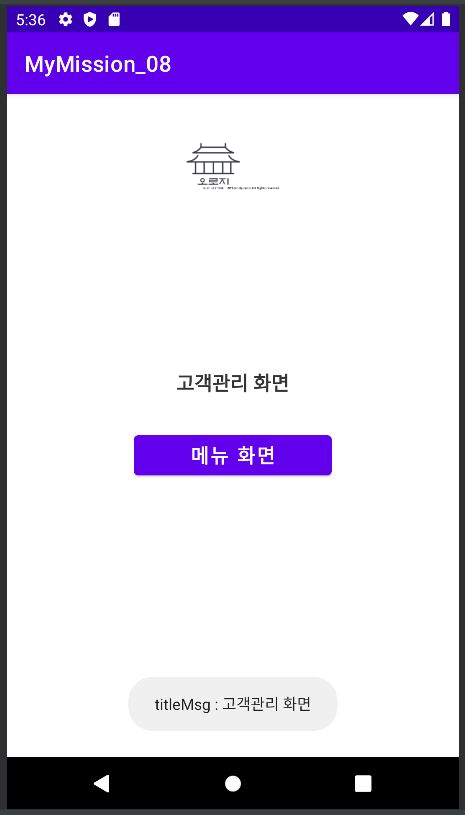
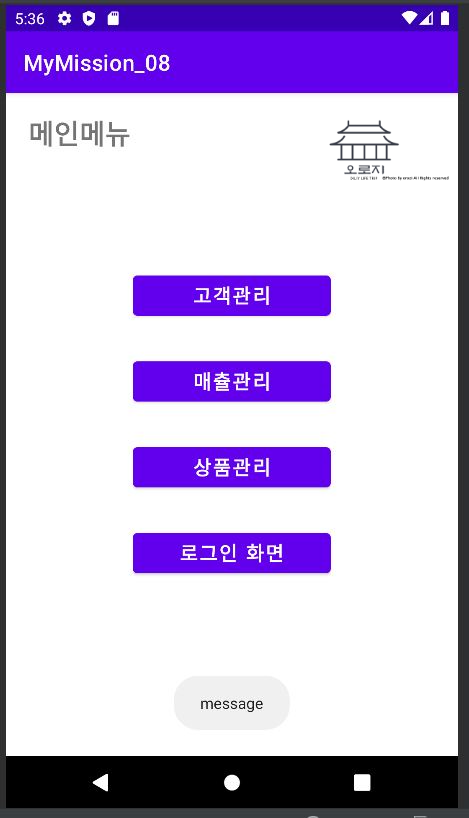
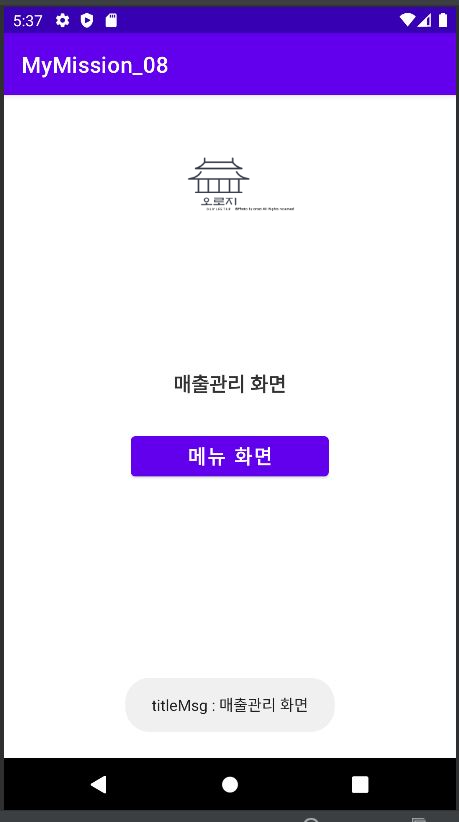
2023.09.13 - [STUDY/안드로이드] - [안드로이드앱] 로그인 화면과 메뉴 화면 전환하기
[안드로이드앱] 로그인 화면과 메뉴 화면 전환하기
로그인 화면과 메뉴 화면 전환하기 (Do it! 안드로이드앱프로그래밍 도전과제07) 대부분의 업무용 앱에서 필요한 로그인 화면과 메뉴 화면을 간단하게 만들고, 두 화면 간을 전환하면서 토스트로
sweet-brown.tistory.com
'STUDY > 안드로이드' 카테고리의 다른 글
[ Android Studio ] 고객정보 입력 화면 구성하기(Do it!안드로이드앱 프로그래밍 도전과제_09) (1) | 2023.11.06 |
---|---|
[안드로이드앱] 로그인 화면과 메뉴 화면 전환하기 (46) | 2023.09.13 |
[안드로이드앱] 시크바와 프로그레스바 보여주기 (67) | 2023.09.10 |
[안드로이드앱] 두 종류의 버튼 모양 만들기 (66) | 2023.09.06 |
[안드로이드앱] SMS 입력화면 만들고 글자의 수 표시하기 (66) | 2023.09.02 |