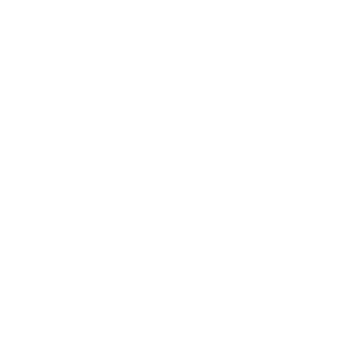
Do it! 안드로이드앱 프로그래밍 도전과제 04
화면 위쪽에 텍스트 입력상자, 아래쪽에 [전송]과 [닫기] 버튼을
수평으로 배치해 보세요
① MS로 문자를 전송하는 화면은 위쪽에 텍스트 입력상자, 아래쪽에 [전송]과 [닫기] 버튼을 수평으로 배치해 보세요
② 텍스트 입력상자 바로 아래에 입력되는 글자의 바이트 수를 '10/80 바이트'와 같은 포맷으로 표시하되 우측 정렬로
하도록 하고 색상을 눈에 잘 띠는 다른 색으로 설정합니다
③ 텍스트 입력상자에 입력되는 글자의 크기와 줄 간격을 조정하여 한 줄에 한글 8글자가 들어가도록 만들어 봅니다
④ [전송] 버튼을 누르면 입력된 글자를 화면에 토스트로 표시하여 내용을 확인할 수 있도록 합니다
※ 참고할 점
화면에서 '10/80 바이트'로 된 글자 부분을 가장 위쪽으로 배치합니다
입력상자에 글자가 입력될 때마다 자동으로 호출되는 메서드를 사용합니다
D1
[ CODE ]
<activity_main.xml>
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<RelativeLayout
android:id="@+id/inputlayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_above="@+id/buttonlayout"
android:layout_alignParentTop="true">
<EditText
android:id="@+id/inputMessage"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_above="@+id/inputCount"
android:layout_alignParentTop="true"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:cacheColorHint="#00000000"
android:gravity="top"
android:listSelector="#00000000"
android:maxLength="80"
android:textSize="48sp"
tools:ignore="SpeakableTextPresentCheck" />
<TextView
android:id="@+id/inputCount"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_alignParentBottom="true"
android:layout_marginTop="10dp"
android:layout_marginRight="10dp"
android:layout_marginBottom="10dp"
android:text="0/80 바이트"
android:textColor="#ffff00ff"
android:textSize="18sp" />
</RelativeLayout>
<LinearLayout
android:id="@+id/buttonlayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:orientation="vertical">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:orientation="horizontal">
<Button
android:id="@+id/sendButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:paddingLeft="20dp"
android:paddingRight="20dp"
android:text="전송"
android:textSize="18sp" />
<Button
android:id="@+id/closeButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:paddingLeft="20dp"
android:paddingRight="20dp"
android:textSize="18sp"
android:text="닫기" />
</LinearLayout>
</LinearLayout>
</RelativeLayout>
<MainActivity.java>
package com.example.mission04;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.text.Editable;
import android.text.TextWatcher;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import java.io.UnsupportedEncodingException;
public class MainActivity extends AppCompatActivity {
EditText inputMessage;
TextView inputCount;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
inputMessage = findViewById(R.id.inputMessage);
inputCount = findViewById(R.id.inputCount);
Button sendButton = findViewById(R.id.sendButton);
sendButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String message = inputMessage.getText().toString();
Toast.makeText(getApplicationContext(), "전송할 메시지\n\n" + message, Toast.LENGTH_LONG).show();
}
});
Button closeButton = findViewById(R.id.closeButton);
closeButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
finish();
}
});
TextWatcher watcher = new TextWatcher() {
public void onTextChanged(CharSequence str, int start, int before, int count) {
byte[] bytes = null;
try {
bytes = str.toString().getBytes("KSC5601");
int strCount = bytes.length;
inputCount.setText(strCount + " / 80바이트");
} catch(UnsupportedEncodingException ex) {
ex.printStackTrace();
}
}
public void beforeTextChanged(CharSequence s, int start, int count, int after) {
}
public void afterTextChanged(Editable strEditable) {
String str = strEditable.toString();
try {
byte[] strBytes = str.getBytes("KSC5601");
if(strBytes.length > 80) {
strEditable.delete(strEditable.length()-2, strEditable.length()-1);
}
} catch(Exception ex) {
ex.printStackTrace();
}
}
};
inputMessage.addTextChangedListener(watcher);
}
}
https://sweet-brown.tistory.com/2
[Do it!안드로이드앱 프로그래밍] 도전미션_03
두 개의 이미지뷰를 한 화면에 보여주고 하나의 이미지를 두 개의 이미지뷰에서 번갈아 보여주도록 만들어 보세요 ① 화면을 위와 아래 두 영역으로 나누고 그 영역에 각각 이미지뷰를 배치합
sweet-brown.tistory.com
D2
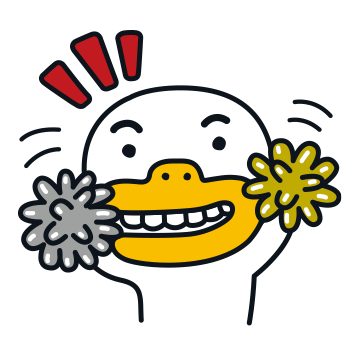
'STUDY > 안드로이드' 카테고리의 다른 글
[Android] Do it! 안드로이드 도전08세 개 이상의 화면 만들어 전환하기 (0) | 2023.09.16 |
---|---|
[안드로이드앱] 로그인 화면과 메뉴 화면 전환하기 (46) | 2023.09.13 |
[안드로이드앱] 시크바와 프로그레스바 보여주기 (67) | 2023.09.10 |
[안드로이드앱] 두 종류의 버튼 모양 만들기 (66) | 2023.09.06 |
[Do it!안드로이드앱 프로그래밍] 도전미션_03 (29) | 2023.09.01 |